Summary: in this tutorial, you’ll learn how to develop the Hello World program in TypeScript.
TypeScript Hello World program in node.js
First, create a new directory to store the code, e.g., helloworld
.
Second, launch VS Code and open that directory.
Third, create a new TypeScript file called app.ts
. The extension of a TypeScript file is .ts
.
Fourth, type the following source code in the app.ts
file:
let message: string = 'Hello, World!';
console.log(message);
Code language: JavaScript (javascript)
Fifth, launch a new Terminal within the VS Code by using the keyboard shortcut Ctrl+`
or follow the menu Terminal > New Terminal
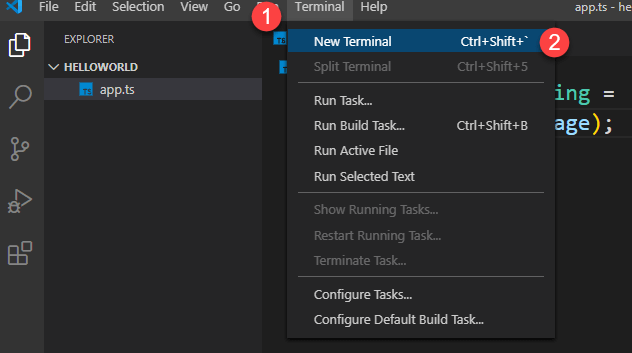
Sixth, type the following command on the Terminal to compile the app.ts
file:
tsc app.ts
Code language: CSS (css)
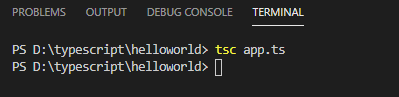
If everything is fine, you’ll see a new file called app.js
is generated by the TypeScript compiler:
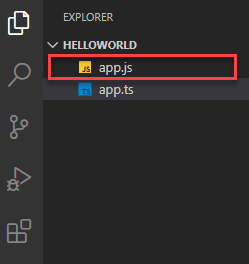
To run the app.js
file in node.js
, you use the following command:
node app.js
Code language: CSS (css)
If you installed the ts-node
module mentioned in the setting up TypeScript development environment, you can use just one command to compile the TypeScript file and execute the output file in one shot:
ts-node app.ts
Code language: CSS (css)
TypeScript Hello World program in Web Browsers
The following steps show you how to create a webpage that shows the Hello, World!
message on web browsers.
First, create a new file called index.html
and include the app.js
as follows:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>TypeScript: Hello, World!</title>
</head>
<body>
<script src="app.js"></script>
</body>
</html>
Code language: HTML, XML (xml)
Second, change the app.ts
code to the following:
let message: string = 'Hello, World!';
// create a new heading 1 element
let heading = document.createElement('h1');
heading.textContent = message;
// add the heading the document
document.body.appendChild(heading);
Code language: JavaScript (javascript)
Third, compile the app.ts
file:
tsc app.ts
Code language: CSS (css)
Fourth, open the Live Server from the VS code by right-mouse click the index.html and select the Open with Live Server option:
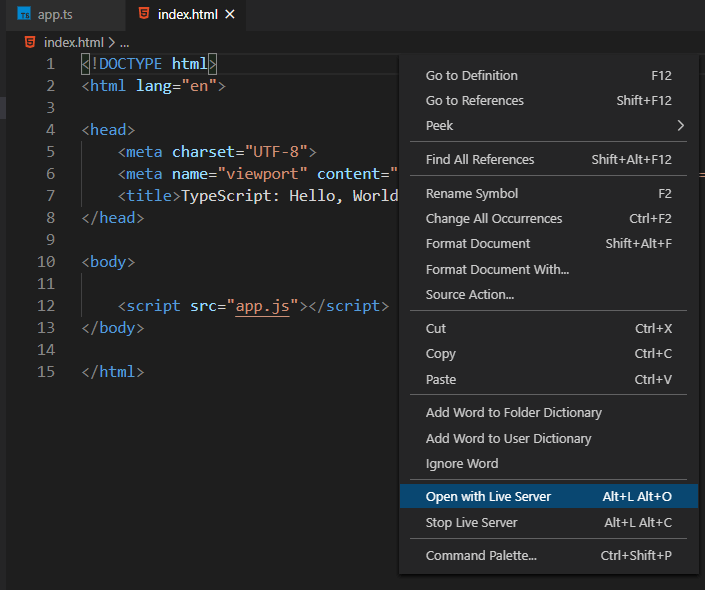
The Live Server will open the index.html
with the following message:
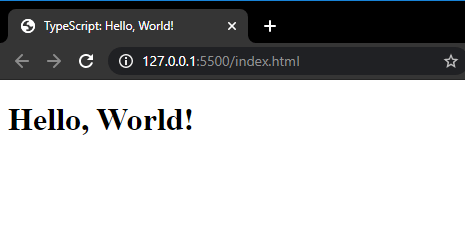
To make the changes, you need to edit the app.ts
file. For example:
let message: string = 'Hello, TypeScript!';
let heading = document.createElement('h1');
heading.textContent = message;
document.body.appendChild(heading);
Code language: JavaScript (javascript)
And compile the app.ts
file:
tsc app.ts
Code language: CSS (css)
The TypeScript compiler will generate a new app.js
file, and the Live Server will automatically reload it on the web browser.
Note that the app.js
is the output file of the app.ts
file, therefore, you should never directly change the code in this file, or you’ll lose the changes once you recompile the app.ts
file.
In this tutorial, you have learned how to create the first program in TypeScript called Hello, World!
that works on node.js and web browsers.